Scikit-Learn’s preprocessing module offers a wide range of tools to prepare and preprocess data for machine learning tasks. One of these tools is the label_binarize function, which plays a crucial role in transforming categorical labels into binary format. Let’s dive into what this function is all about and how it can be useful in machine learning workflows.

What is label_binarize?
The label_binarize function is a part of Scikit-Learn’s preprocessing module. It is designed to convert categorical labels into a binary format suitable for machine learning algorithms. This function is particularly useful for tasks where multiple classes need to be transformed into a binary representation, such as in one-vs-all classification.
How Does label_binarize Work?
The label_binarize function takes as input an array of categorical labels and a list of classes. It then transforms the labels into a binary matrix, where each column represents a class and each row represents a sample. The function assigns a value of 1 to the corresponding class column for each sample, and 0 to all other columns.
Why Use label_binarize?
The label_binarize function is a valuable tool for transforming categorical labels into a format suitable for machine learning algorithms. It allows you to convert multi-class problems into binary classification problems, which can simplify the task of training models and making predictions. This function is commonly used in scenarios where algorithms require binary labels, such as in certain types of classifiers and evaluation metrics.
How to Use label_binarize?
Using the label_binarize function is straightforward. Simply provide the array of categorical labels and the list of classes as input. The function will return the binary matrix representation of the labels. It’s important to note that the class order in the binary matrix matches the order of the classes provided in the input.
Example Use Case
Imagine you have a classification task with three classes: “cat,” “dog,” and “bird.” By using the label_binarize function, you can transform these categorical labels into binary representations where each row corresponds to a sample and each column corresponds to a class. This allows you to train binary classifiers individually for each class, effectively solving a multi-class problem as a series of binary classification tasks.
Benefits of label_binarize
- Converts categorical labels into a binary matrix format.
- Useful for multi-class to binary transformation.
- Simplifies training of binary classifiers.
- Compatible with various machine learning algorithms and evaluation metrics.
Python Code Examples
Example 1: Binary Classification
import numpy as np
from sklearn.preprocessing import label_binarize
#Original categorical labels
labels = ['cat', 'dog', 'cat', 'dog', 'bird']
#Binarize the labels
binarized_labels = label_binarize(labels, classes=['cat', 'dog'])
print('Labels:\n',labels)
print('Binarized:\n',binarized_labels)
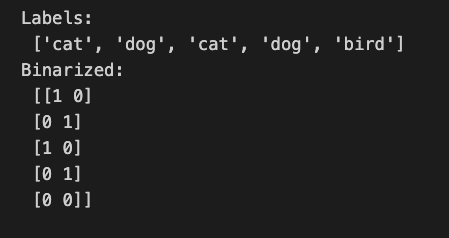
Example 2: Multi-Class Classification
import numpy as np
from sklearn.preprocessing import label_binarize
#Original categorical labels
labels = ['red', 'green', 'blue', 'green', 'red']
#Binarize the labels
binarized_labels = label_binarize(labels, classes=['red', 'green', 'blue'])
print('Labels:\n',labels)
print('Binarized:\n',binarized_labels)

Visualize Label_binarize with Python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.preprocessing import label_binarize
from sklearn.datasets import load_iris
# Load the Iris dataset
iris = load_iris()
y = iris.target
# Binarize the labels
binarized_labels = label_binarize(y, classes=[0, 1, 2])
# Create a scatter plot for each class
for class_idx in range(binarized_labels.shape[1]):
plt.scatter(iris.data[:, 0], iris.data[:, 1], c=binarized_labels[:, class_idx], label=f'Class {class_idx}')
plt.xlabel(iris.feature_names[0])
plt.ylabel(iris.feature_names[1])
plt.title('Effects of label_binarize on Data Distribution')
plt.legend()
plt.show()

Important Concepts in Scikit-Learn Preprocessing label_binarize
- Data Binarization
- Multi-class Classification
- One-vs-All Strategy
- Multi-label Classification
- Binary Classification
- Label Encoding
To Know Before You Learn Scikit-Learn Preprocessing label_binarize
- Basic understanding of classification problems in machine learning.
- Familiarity with binary classification and multi-class classification concepts.
- Knowledge of encoding categorical variables.
- Understanding of label encoding and one-hot encoding techniques.
- Awareness of multi-label classification scenarios.
- Familiarity with the basics of data preprocessing in machine learning.
What’s Next?
After learning about Scikit-Learn Preprocessing label_binarize, you might find it beneficial to explore the following topics in machine learning:
- Further exploration of data preprocessing techniques, such as scaling, imputation, and feature engineering.
- Introduction to various classification algorithms, such as decision trees, random forests, support vector machines, and neural networks.
- Understanding model evaluation metrics for classification tasks, including accuracy, precision, recall, F1-score, and ROC curves.
- Advanced topics in multi-label classification and handling imbalanced datasets.
- Exploring other preprocessing techniques in Scikit-Learn, such as scaling, normalization, and feature selection.
Relevant Entities
Entity | Properties |
---|---|
label_binarize | Converts categorical labels into binary matrix format for machine learning. |
Categorical Labels | Non-numeric class labels used for classification tasks. |
Binary Matrix | Matrix representation of labels with binary values (0 or 1). |
Classes | List of unique classes present in the categorical labels. |
Multi-Class Problems | Classification tasks involving more than two classes. |
Binary Classification | Classification tasks with two possible outcomes (positive or negative). |
Sources
scikit-learn.org/stable/modules/generated/sklearn.preprocessing.label_binarize.html">Scikit-Learn Documentation
Conclusion
The label_binarize function in Scikit-Learn’s preprocessing module provides a powerful tool for transforming categorical labels into binary representations. It enables the conversion of multi-class problems into a binary classification format, making it easier to apply machine learning algorithms and evaluation metrics. Incorporating label_binarize into your preprocessing pipeline can enhance the efficiency and effectiveness of your machine learning workflows.