Non-linear transformation is a feature transformation technique used to transform the data into a non-linear space, where the patterns are more easily discernible. In this article, we will explore the concept of non-linear transformation and its applications in machine learning.
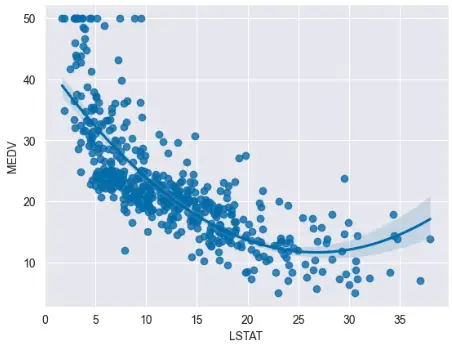
What is Non-linear Transformation?
Non-linear transformation is a technique used to transform the input features into a non-linear space. It is based on the idea that in some cases, the patterns in the data are more easily discernible in a non-linear space than in the original space. Non-linear transformation is achieved by applying a non-linear function to the input features. The transformed features are then used as input to the machine learning algorithm.
Why Non-linear Transformation?
Linear models are simple and easy to interpret, but they are limited in their ability to capture complex patterns in the data. Non-linear transformation can help overcome this limitation by transforming the input features into a space where the patterns are more easily discernible. Non-linear transformation is especially useful when dealing with highly non-linear data, such as images, audio, and text.
Types of Non-linear Transformation
There are several types of non-linear transformation, including polynomial transformation, log transformation, and exponential transformation. Polynomial transformation is a common non-linear transformation technique that involves transforming the input features into a polynomial space. Log transformation is another common non-linear transformation technique that involves taking the logarithm of the input features. Exponential transformation involves transforming the input features using an exponential function.
Applications of Non-linear Transformation
Non-linear transformation is used in various applications of machine learning, such as image recognition, speech recognition, and natural language processing. In image recognition, non-linear transformation is used to transform the image pixels into a non-linear space where the patterns in the image are more easily discernible. In speech recognition, non-linear transformation is used to transform the audio signal into a non-linear space where the speech patterns are more easily discernible. In natural language processing, non-linear transformation is used to transform the text into a non-linear space where the patterns in the text are more easily discernible.
Challenges of Non-linear Transformation
Non-linear transformation can be computationally expensive, especially when dealing with high-dimensional data. The choice of the non-linear function used for transformation is also important, as some functions may perform better than others on different types of data. Overfitting can also be a challenge when using non-linear transformation, as the transformed features may capture noise in the data instead of the underlying patterns.
Python code Examples
Non-linear transformation on Boston Housing Dataset
from sklearn.datasets import load_boston
from sklearn.preprocessing import PowerTransformer
import seaborn as sns
# Load the Boston Housing dataset
boston_dataset = load_boston()
# Extract the features and target variable
X = boston_dataset.data
y = boston_dataset.target
# Convert the dataset to a pandas dataframe
boston_df = pd.DataFrame(boston_dataset.data, columns=boston_dataset.feature_names)
# Add a new column for the target variable
boston_df['MEDV'] = boston_dataset.target
# Visualize non-linear relationship between LSTAT and MEDV using regplot
sns.regplot(x='LSTAT', y='MEDV', data=boston_df, order=2)
# Perform Non-linear transformation using PowerTransformer
pt = PowerTransformer()
X_transformed = pt.fit_transform(X)
In this example, we first load the Boston Housing dataset and extract the features and target variable. We then perform Non-linear transformation on the features using the PowerTransformer from the scikit-learn library. The transformed features are stored in the X_transformed variable.

Useful Python Libraries for Non-linear transformation
- NumPy: exp, power, log
- SciPy: expit, tanh
- scikit-learn: preprocessing.PowerTransformer, preprocessing.QuantileTransformer, preprocessing.KernelCenterer, preprocessing.FunctionTransformer
The NumPy library provides various mathematical functions such as exponential, power, and logarithmic functions that can be used for Non-linear transformation.
The SciPy library offers activation functions such as expit and tanh, which can be used in Non-linear transformation.
The scikit-learn library provides several transformers such as PowerTransformer, QuantileTransformer, KernelCenterer, and FunctionTransformer that can perform Non-linear transformation on the input data.
Datasets useful for Non-linear transformation
Boston Housing Dataset
from sklearn.datasets import load_boston
#Load the Boston Housing dataset
boston_dataset = load_boston()
#Extract the features and target variable
X = boston_dataset.data
y = boston_dataset.target
The Boston Housing dataset is a popular regression dataset that contains information about different houses in Boston. The goal is to predict the median value of owner-occupied homes in $1000s. This dataset is useful for learning how to apply Non-linear transformation to continuous variables such as house prices.
MNIST Dataset
from sklearn.datasets import fetch_openml
#Load the MNIST dataset
mnist_dataset = fetch_openml('mnist_784')
#Extract the features and target variable
X = mnist_dataset.data
y = mnist_dataset.target
The MNIST dataset is a popular classification dataset that consists of handwritten digits. The goal is to predict the digit (0-9) based on the image of the handwritten digit. This dataset is useful for learning how to apply Non-linear transformation to image data.
To Know Before You Learn Non-linear transformation?
- Linear algebra (e.g., matrix operations, eigendecomposition)
- Calculus (e.g., derivatives, integrals)
- Basic statistics (e.g., mean, standard deviation)
- Familiarity with Python programming language
- Understanding of linear regression and logistic regression
- Knowledge of scikit-learn library
Important Concepts in Non-linear transformation
- Linear vs Non-linear Transformations
- Polynomial Features
- Kernel Methods
- Activation Functions
- Neural Networks
- Feature Scaling
What’s Next?
- Regularization methods (e.g., L1, L2)
- Gradient descent optimization algorithms
- Support Vector Machines (SVM)
- Decision Trees and Random Forests
- Deep Learning (e.g., Artificial Neural Networks, Convolutional Neural Networks)
- Ensemble methods (e.g., Boosting, Bagging)
Relevant entities
Entity | Properties |
---|---|
Non-linear transformation | input data, output data, non-linear function |
Non-linear function | activation function, transfer function, threshold function |
Neural network | input layer, hidden layer, output layer, weights |
Support vector machine (SVM) | kernel function, support vectors, hyperplane |
Decision tree | root node, internal node, leaf node, splitting criteria |
Regression | dependent variable, independent variable, curve fitting |
Frequently Asked Questions
What is non-linear transformation?
Input to output mapping
What is a non-linear function?
Activation/transfer/threshold func
What is a neural network?
Input/hidden/output layers
What is a support vector machine (SVM)?
Kernel/sv/hyperplane
What is a decision tree?
Root/internal/leaf node
What is regression?
Dependent/independent variable
Sources
- Nonlinear Feature Transformation: https://scikit-learn.org/stable/modules/preprocessing.html#non-linear-transformation
- Non-linear Transformations: https://towardsdatascience.com/non-linear-transformations-8f6b421ce0a6
- Non-Linear Transformations and Feature Engineering for Machine Learning: https://towardsdatascience.com/non-linear-transformations-and-feature-engineering-for-machine-learning-792c96a9b2b2
- Non-linear transformation: https://en.wikipedia.org/wiki/Non-linear_transformation
- Feature Transformation: Non-linear to Linear: https://www.datacamp.com/community/tutorials/feature-transformation-non-linear-to-linear
Conclusion
Non-linear transformation is a powerful technique used in machine learning to transform the input features into a non-linear space, where the patterns in the data are more easily discernible. It is especially useful when dealing with highly non-linear data, such as images, audio, and text. There are several types of non-linear transformation, including polynomial transformation, log transformation, and exponential transformation. Non-linear transformation is used in various applications of machine learning, such as image recognition, speech recognition, and natural language processing. However, it also comes with challenges, such as computational expense and overfitting. Understanding the power and limitations of non-linear transformation is important for building effective machine learning models.