Sentiment analysis, also known as opinion mining, is a subfield of natural language processing (NLP) that helps us understand the emotions and opinions expressed in text data. In this article, we will dive deeper into the topic of sentiment analysis and explore its applications and techniques.
What is Sentiment Analysis
“Sentiment analysis is the use of natural language processing, text analysis, and computational linguistics to identify and extract subjective information from textual sources.”
– Wikipedia
In simpler terms, sentiment analysis is the process of using machine learning algorithms to identify and extract the emotional content from text data. It involves analyzing a piece of text and determining whether the expressed opinion is positive, negative, or neutral.
Techniques of Sentiment Analysis
Sentiment analysis can be performed using various techniques, ranging from rule-based methods to machine learning algorithms.
Rule-based methods involve creating a set of rules or heuristics to identify the sentiment of a text. These rules can be based on the presence of certain words or phrases that indicate a positive or negative sentiment.
Machine learning algorithms, on the other hand, involve training a model on a large dataset of labeled text data. The model learns to identify the sentiment of a text based on the patterns and relationships in the data.
Sentiment Analysis in Python (with NLTK)
To perform sentiment analysis in Python, the nltk
library offers the sentiment.SentimentIntensityAnalyzer class. In this code example, we use the Matplotlib library to create a horizontal bar chart, where the sentiment labels are shown on the y-axis and the sentiment values are shown on the x-axis. The sentiment score is indicated by a red dashed line on the chart. The chart provides a visual representation of the sentiment analysis result for the text.
import nltk
import matplotlib.pyplot as plt
from nltk.sentiment import SentimentIntensityAnalyzer
nltk.download('vader_lexicon')
sia = SentimentIntensityAnalyzer()
text = "I love spending time with my friends and family!"
sentiment = sia.polarity_scores(text)
# Extract the sentiment score
score = sentiment['compound']
# Define the bar chart labels and values
labels = ['Negative', 'Neutral', 'Positive']
values = [sentiment['neg'], sentiment['neu'], sentiment['pos']]
# Create a horizontal bar chart
plt.barh(labels, values)
# Add a vertical line to indicate the sentiment score
plt.axvline(x=score, color='r', linestyle='--')
# Add labels and title
plt.xlabel('Sentiment Score')
plt.ylabel('Sentiment')
plt.title('Sentiment Analysis Result')
# Display the chart
plt.show()
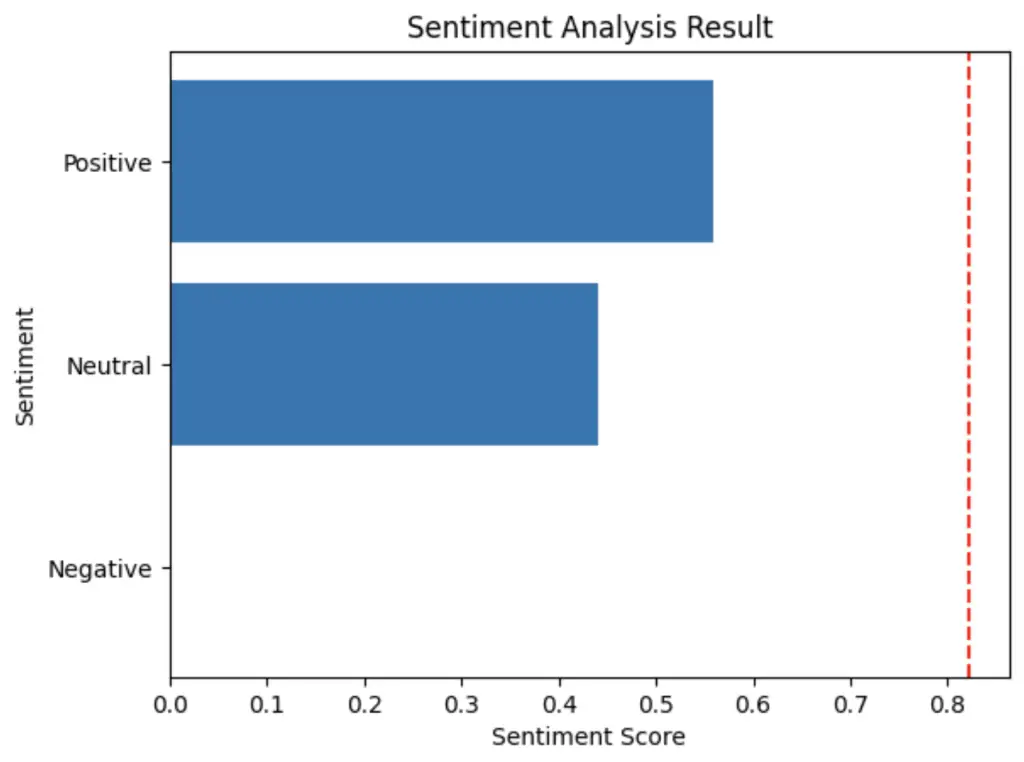
Sentiment Analysis with SpaCy and TextBlob
It is possible to perform sentiment analysis in Python using the SpaCy and the TextBlob libraries.
Here, we have two sentences that we will try to analyze their sentiments using SpaCy and TextBlob.
The higher the score, the more positive the sentiment is.
import spacy
from textblob import TextBlob
import matplotlib.pyplot as plt
# Load spaCy model
nlp = spacy.load("en_core_web_sm")
# Input texts
text1 = "I love spending time with my friends and family!"
text2 = "Programming can be challenging, but it's also rewarding."
# Process the texts using spaCy
doc1 = nlp(text1)
doc2 = nlp(text2)
# Analyze sentiment using TextBlob
sentiment1 = TextBlob(text1).sentiment.polarity
sentiment2 = TextBlob(text2).sentiment.polarity
# Display the sentiment scores
print(f"Sentiment Score for Text 1: {sentiment1:.2f}")
print(f"Sentiment Score for Text 2: {sentiment2:.2f}")
# Visualize sentiment scores
fig, ax = plt.subplots()
ax.bar(["Text 1", "Text 2"], [sentiment1, sentiment2], color=['skyblue', 'lightcoral'])
ax.set_ylabel('Sentiment Score')
ax.set_title('Text Sentiment Analysis')
# Show the plot
plt.show()
print("\n" + "="*40)
print(f"\nText Analysis of doc1:")
for sentence in doc1.sents:
print(f" - Sentence: {sentence.text}")
for token in sentence:
print(f" - Token: {token.text}, POS: {token.pos_}, Lemma: {token.lemma_}")
print("\n" + "="*40)
print(f"\nText Analysis of doc2:")
for sentence in doc2.sents:
print(f" - Sentence: {sentence.text}")
for token in sentence:
print(f" - Token: {token.text}, POS: {token.pos_}, Lemma: {token.lemma_}")
Sentiment Score for Text 1: 0.62
Sentiment Score for Text 2: 0.50

Example of how SpaCy interpreted both sentences.
========================================
Text Analysis of doc1:
- Sentence: I love spending time with my friends and family!
- Token: I, POS: PRON, Lemma: I
- Token: love, POS: VERB, Lemma: love
- Token: spending, POS: VERB, Lemma: spend
- Token: time, POS: NOUN, Lemma: time
- Token: with, POS: ADP, Lemma: with
- Token: my, POS: PRON, Lemma: my
- Token: friends, POS: NOUN, Lemma: friend
- Token: and, POS: CCONJ, Lemma: and
- Token: family, POS: NOUN, Lemma: family
- Token: !, POS: PUNCT, Lemma: !
========================================
Text Analysis of doc2:
- Sentence: Programming can be challenging, but it's also rewarding.
- Token: Programming, POS: NOUN, Lemma: programming
- Token: can, POS: AUX, Lemma: can
- Token: be, POS: AUX, Lemma: be
- Token: challenging, POS: VERB, Lemma: challenge
- Token: ,, POS: PUNCT, Lemma: ,
- Token: but, POS: CCONJ, Lemma: but
- Token: it, POS: PRON, Lemma: it
- Token: 's, POS: AUX, Lemma: be
- Token: also, POS: ADV, Lemma: also
- Token: rewarding, POS: ADJ, Lemma: rewarding
- Token: ., POS: PUNCT, Lemma: .
Datasets useful for Sentiment analysis
IMDb Movie Reviews
# Python example using NLTK
from nltk.corpus import movie_reviews
#Load positive and negative reviews
positive_reviews = [movie_reviews.raw(fileid) for fileid in movie_reviews.fileids('pos')]
negative_reviews = [movie_reviews.raw(fileid) for fileid in movie_reviews.fileids('neg')]
#Combine positive and negative reviews
reviews = [(review, 'pos') for review in positive_reviews] + [(review, 'neg') for review in negative_reviews]
reviews
Useful Python Libraries for Sentiment analysis
- nltk: VADER, Naive Bayes, Maximum Entropy
- spaCy: Rule-based matching, TextCategorizer
- TextBlob: PatternAnalyzer, NaiveBayesAnalyzer
- Scikit-learn: Logistic Regression, Random Forest, Support Vector Machines (SVM)
- TensorFlow: Convolutional Neural Networks (CNNs), Long Short-Term Memory (LSTM) networks, Recursive Neural Networks (RNNs)
- Keras: LSTM, Convolutional Neural Networks (CNNs), Recurrent Neural Networks (RNNs)
Challenges of Sentiment Analysis
Despite its wide range of applications, sentiment analysis faces various challenges. One of the major challenges is sarcasm and irony in text. It is difficult for machines to identify the intended sentiment behind sarcastic or ironic statements.
Another challenge is the context of the text. The same piece of text can have a different sentiment depending on the context in which it is used. Machines find it challenging to understand the nuances of the language and the context in which it is used.
Important Concepts in Sentiment analysis
- Natural Language Processing (NLP)
- Text Preprocessing Techniques
- Feature Extraction Methods
- Supervised Learning Algorithms
- Unsupervised Learning Algorithms
- Deep Learning Models
- Word Embeddings
- Sentiment Lexicons
- Evaluation Metrics
- Overfitting and Underfitting
- Bias and Fairness in Sentiment analysis
To Know Before You Learn Sentiment analysis?
- Basics of Python Programming
- Familiarity with Numpy, Pandas, and Matplotlib libraries
- Fundamentals of Machine Learning
- Concepts of Supervised and Unsupervised Learning
- Text Preprocessing Techniques
- Basic knowledge of Natural Language Processing (NLP) and Linguistics
- Feature Engineering Methods
- Understanding of Evaluation Metrics
- Familiarity with Deep Learning Models
- Experience with Jupyter Notebook or other Python IDEs
What’s Next?
- Text Classification
- Topic Modelling
- Named Entity Recognition (NER)
- Text Summarization
- Machine Translation
- Speech Recognition
- Chatbot Development
- Recommendation Systems
- Customer Feedback Analysis
- Social Media Analytics
Relevant entities
Entity | Properties |
---|---|
Sentiment analysis | Subfield of natural language processing that helps us understand the emotions and opinions expressed in text data. |
Natural Language Processing (NLP) | A field of computer science and artificial intelligence that deals with the interaction between computers and humans in natural language. |
Machine Learning Algorithms | Used to identify and extract the emotional content from text data in sentiment analysis. |
Rule-based methods | Techniques for sentiment analysis that involve creating a set of rules or heuristics to identify the sentiment of a text. |
Supervised learning | A type of machine learning where a model is trained on labeled data to predict the sentiment of a text. |
Unsupervised learning | A type of machine learning where a model is trained on unlabeled data to identify patterns and relationships in the data. |
Sarcasm and irony | Challenges in sentiment analysis due to their difficulty for machines to identify the intended sentiment behind sarcastic or ironic statements. |
Context | A challenge in sentiment analysis where the same piece of text can have a different sentiment depending on the context in which it is used. |
Conclusion
In conclusion, sentiment analysis is a powerful tool that helps us understand the emotions and opinions expressed in text data. It has a wide range of applications and can be performed using various techniques. However, it also faces various challenges that need to be addressed. With the advancements in machine learning and NLP, we can expect sentiment analysis to become more accurate and reliable in the future.
Sources
- “A Comprehensive Guide to Sentiment Analysis” by MonkeyLearn
- “Sentiment Analysis: A Definitive Guide” by Algorithmia
- “What Is Sentiment Analysis? A
In conclusion, sentiment analysis is a powerful tool that helps us understand the emotions and opinions expressed in text data. It has a wide range of applications and can be performed using various techniques. However, it also faces various challenges that need to be addressed. With the advancements in machine learning and NLP, we can expect sentiment analysis to become more accurate and reliable in the future. Complete Guide to Opinion Mining” by Brandwatch - “Sentiment Analysis with Machine Learning: A Complete Guide” by MonkeyLearn
- “Introduction to Sentiment Analysis in Python” by Towards Data Science
- “Sentiment Analysis: Concept, Analysis and Applications” by IEEE Xplore
- “Sentiment Analysis in Machine Learning: Everything You Need to Know” by Analytics India Magazine
- “The Ultimate Guide to Sentiment Analysis” by TextRazor
- “Sentiment Analysis in Machine Learning: A Beginner’s Guide” by Data Science Central
- “How to Perform Sentiment Analysis in Python” by Machine Learning Mastery